Chapter 3: Java - Part 4
Of course, when the user hits addDinerButton, we also need the bill splitting part of the app to update and show the new diner. So let's add that functionality. Using the same methodology as before, we'll just copy over the format properties from Views we already made in XML. Import code for TextViews:
import android.widget.TextView;
Then declare the following TextView variables:
private TextView textSplit1;
private TextView textSplit1Dollar;
Then assign them to the TextViews from the bill splitting portion of our app's UI:
textSplit1 = (TextView) findViewById(R.id.textSplit1);
textSplit1Dollar = (TextView) findViewById(R.id.textSplit1Dollar);
Now, add the following to addDinerButton's onClick code block:
TableRow row2 = new TableRow(this);
TextView tv1 = new TextView(this);
tv1.setText(et1.getText().toString());
tv1.setGravity(textSplit1.getGravity());
TextView tv2 = new TextView(this);
tv2.setText(et2.getText().toString());
tv2.setGravity(textSplit1Dollar.getGravity());
row2.addView(tv1);
row2.addView(tv2);
mainTable.addView(row2, 14);
Here, we're creating another TableRow, row2. We're putting 2 TextViews in it, and use the Gravity from our XML, and the Text from the two EditTexts that were created earlier in the code block (et1 and et2), to set their properties. Note the toString method here. Thing is, getText doesn't actually return a string. The EditText version of getText returns something called an Editable. We won't worry about what an Editable is, just know that it's not a string, and we want to pass a string to setText. To turn the return value, that is, the Editable, into a string, we call the toString method on it. After that, we put row2 into mainTable at row number 14, which is the one right under the first customer in the bill splitting portion of our app. Test the new code to see what happens.
Try hitting addDinerButton and look at the bottom of the app. On the bright side, the first couple times you hit it, everything looks right. But if you hit it a few more times, things end up looking odd. Like this:
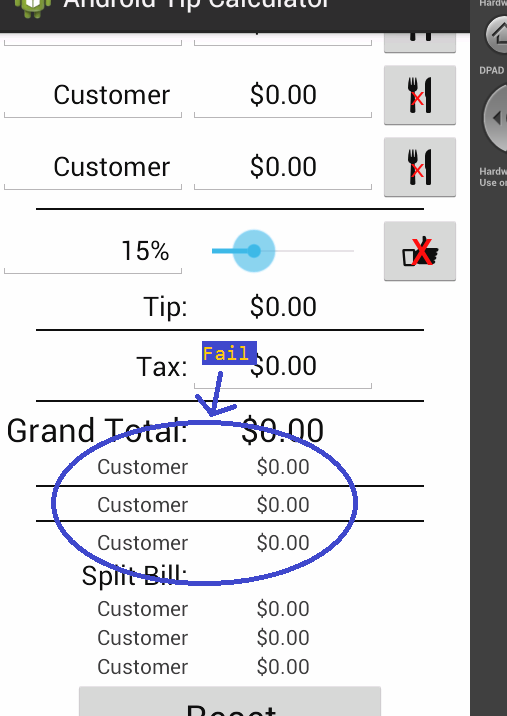
So what's happening here? Well, we're adding the row2 to the 14th row in mainTable. The thing is, whenever we add a new diner, more rows are being created. For example, when we hit addDinerButton for the sixth time, the 14th row in mainTable is by then actually the row directly under Grand Total. So clearly we can't use the constant number 14 as the argument for addView. We need to use an argument that changes based on how many rows are in mainTable. That way, we can guarantee each new diner row is put in mainTable at the right spot. Let's work on that next.
Glossary
getText(): The getText method returns something different depending on what it's called on. The EditText version, for instance, returns an Editable object, that has information about what text was in the EditText.
toString(): The toString method returns a string-ified version of whatever it's called on (assuming it can be string-ified).