Chapter 2: User Interface - Part 1
Now let's open up Eclipse. Then click File, go to New, click Other..., click the triangle next to Android, select Android Application Project, and hit the Next button.
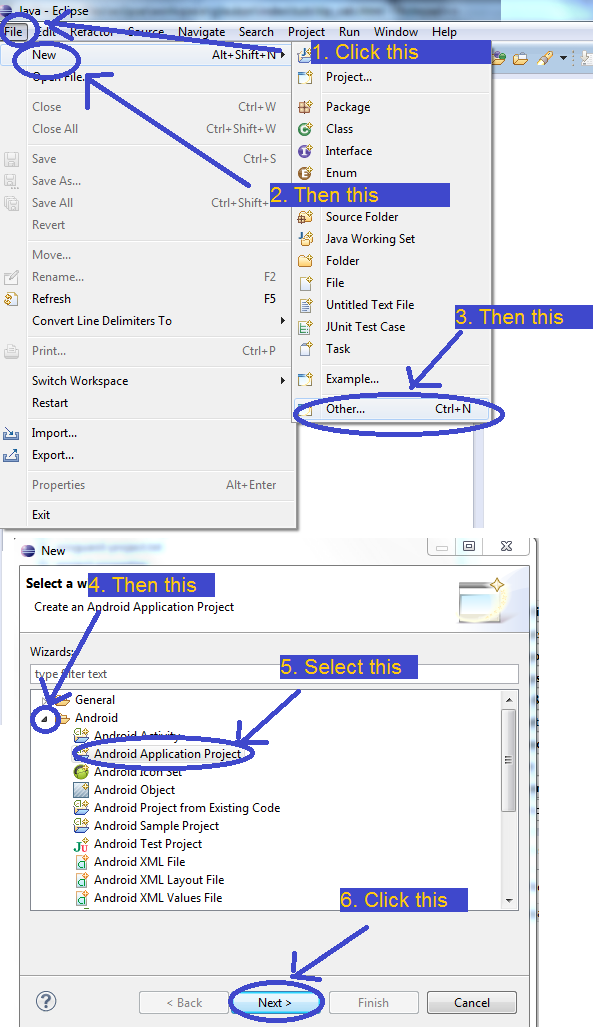
The next few screens will set up your project. The pictures below show what I put in the fields. You can follow along exactly, or change the fields based on your preferences. Most of the fields are very intuitive, but you can also hover over the i icon next to each field for more info. I used Android 2.2 for the Minimum Required SDK, since at the time of this writing (January 2013), using Froyo means you are supporting around 97% of the Android population, which for me, is good enough. If you're reading this tutorial at a much later date than it was written, you can easily check the current distribution of Android versions by googling. Don't worry about the Configure Launcher Icon stuff, we're be replacing the launch icon later.
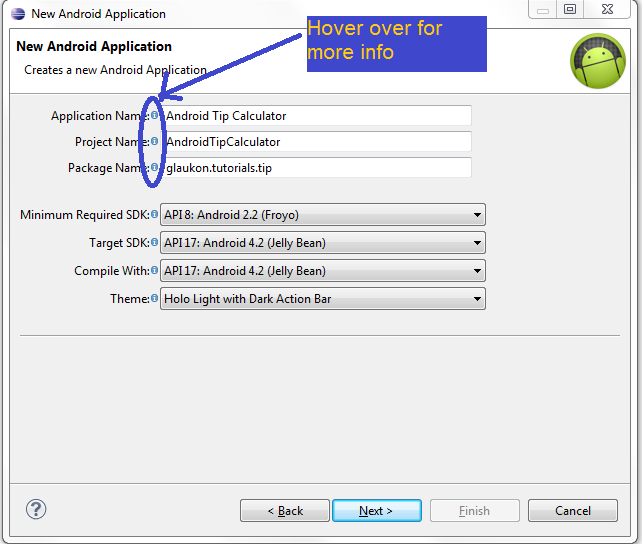
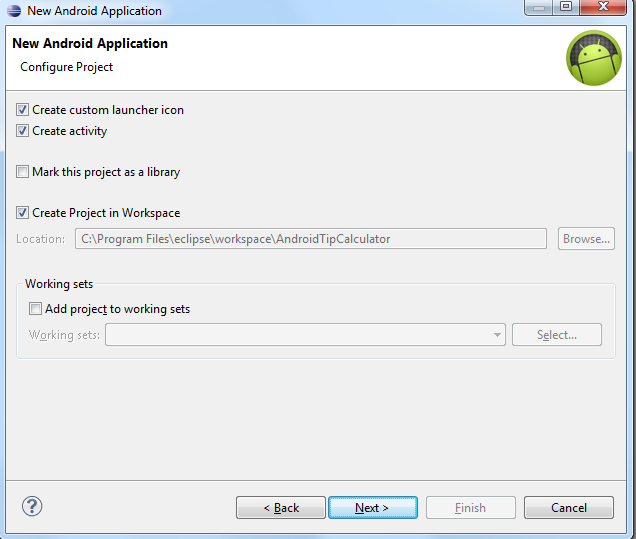
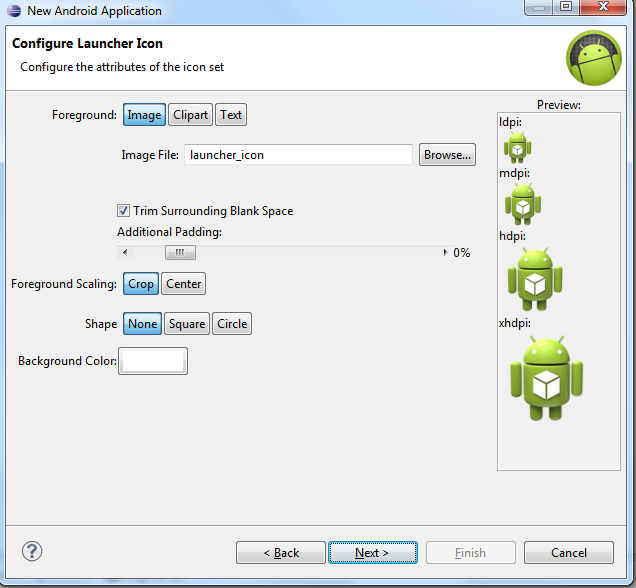
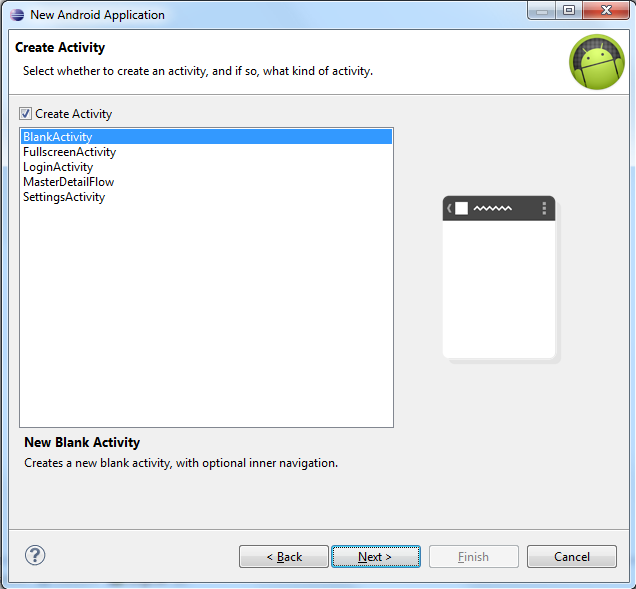
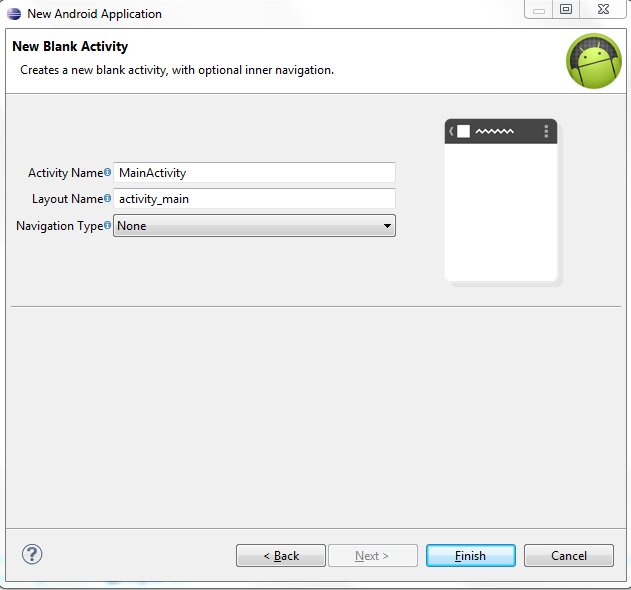
Cool. The main layout XML should now be open. If not, find it in the Package Explorer to the left. It's under res, then layout. The Package Explorer is how Eclipse lets you navigate your project.
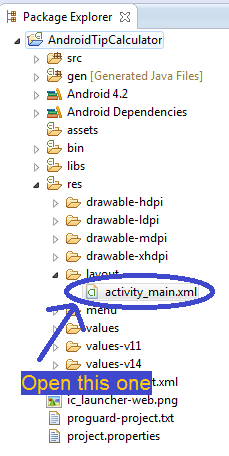
Before moving on, let me talk a little bit about what this XML is. One of the benefits of programming for the Android platform is that we can create our basic UI using XML instead of Java. XML is a much easier language than Java, so this is good. As you can see, Eclipse has automagically created this layout XML file for us, and made it say Hello world!. That's great and all, but useless for us. So let's delete it. Near the bottom, you'll find the Graphical Layout tab, which is currently selected, and the XML tab, named something like activity_main.xml. Click the XML tab, select all the code there, and delete it.
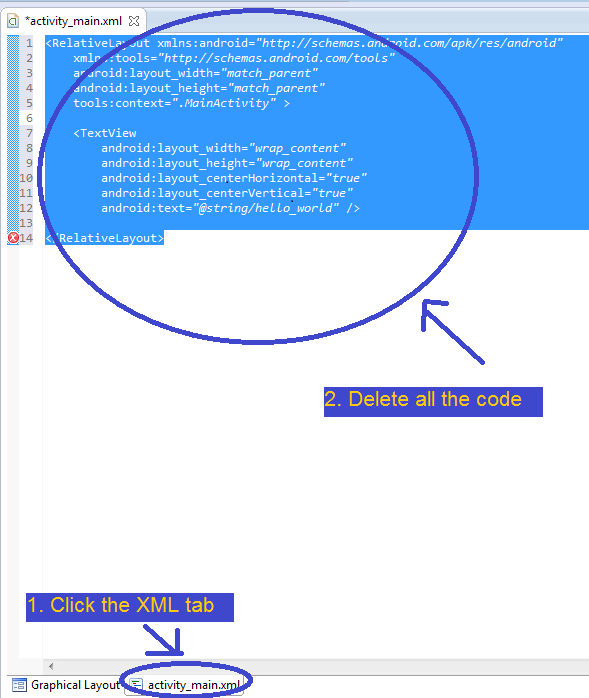
Another cool thing about programming for Android is the fact that we can use the XML tab, or the Graphical Layout tab. The former lets us edit the code directly, when we need to, while the latter lets us quickly create a UI using a drag-and-drop interface. Okay, switch back to the Graphical Layout tab for now. Under the Palette window, click on Composite, then drag and drop ScrollView onto the preview window.
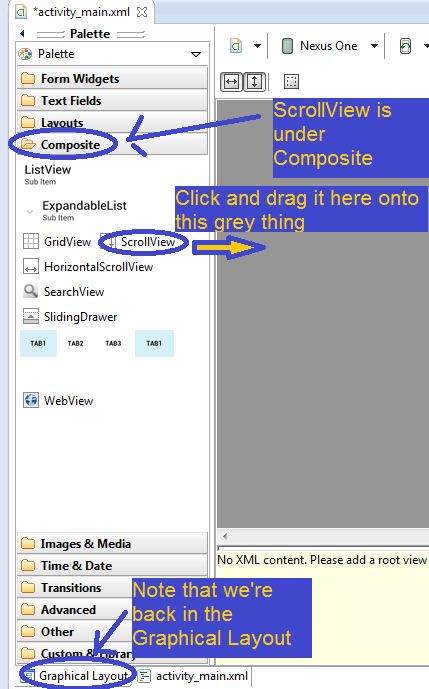
The ScrollView is a View object in Android. This is a tutorial, and not a University course, so I won't go into detail with definitions, but very quickly, an object in programming is a thing that can be changed and manipulated by code. The things that can be changed are its attributes, and the things that you use to change and manupulate it are its methods. You could, for instance, code a cat object, which would have attributes like height and weight, and methods like grow or meow. A View is an object built into Android. It represents something that the end-user sees, and can sometimes interact with. It has attributes like width and height, and lots of built-in methods that come with Android. A ScrollView is a specific kind of View object. It is a View that can be scrolled vertically on Android phones. Generally speaking, if you put all the UI elements (buttons, images, etc.) of your app inside a ScrollView, then if the UI is too large to fit on the screen, it will be scrollable, vertically. A ScrollView has all the attributes and methods that a standard View does, but also its own unique, "scrolly", attributes and methods. In other words, it inherits all the elements of the View object, and then builds on them to make it a different, perhaps upgraded, version of the View object. Here, inheritance refers to the idea of simple objects being the foundation for more complex objects, like how the View object is the foundation for the ScrollView object.
Phew, that was a doozy. Let's move on. If you want more info, the glossary at the bottom has links to more detailed definitions written by far better programmers than myself.
Glossary
Attribute: An attribute is something that describes an object. For instance, string objects in most programming languages have an atrribute called length and refers to how long the string is (i.e. number of characters). If you made an object called 'polygon', you might want to give it attributes like 'number of sides', or 'angle degrees'.
Graphical Layout: It's a drag-and-drop interface in Eclipse that you can use instead of writing straight XML. It's very helpful for beginners that are still just learning XML, but doesn't give you access to all the features that Android has.
Inheritance: Inheritance refers to the way objects can use attributes and methods from other objects. If object A inherits from object B, then object A will have all the attributes and methods object B has. In addition, object A can also have its own unique attributes and methods, thus making it a more particular, niche implementation of object B. Object A can also overwrite the attributes and methods of object B. For example, an object called 'teenager' might inherit from an object called 'mom'. 'Teenager' will inherit attributes like 'max height', 'skin tone', and 'eye color' from 'mom', and methods like 'walk' and 'talk'. However, 'teenager' might have unique attributes like 'acne' and unique methods like 'complain about life'. 'Teenager' might also overwrite the 'walk' attribute so that it looks more like he's strutting because he thinks he owns the world. Or something.
Method: A method is something that an object can do. For example, an object called 'tiger' might have methods like 'run', 'roar', and 'eat people'. Using a method is called 'calling' it (i.e. "I call the 'run' method on 'tiger').
Object: An object in programming is a thing that has attributes and methods. Simple things, like strings and integers, are objects. But there are also much more complicated objects, and you can even make your own.
Package Explorer: The Package Explorer is the window that is, by default, on the left side of Eclipse. It lets you navigate your project, similar to how Windows Explorer lets you navigate your PC.
ScrollView: A ScrollView is a specific kind of View object that allows the elements inside of it to be scrolled vertically.
View: A View is an Android object that, generally speaking, can be seen and sometimes manipulated by the user. It is the basic building block of the user interface of all Android apps. Things like text, images, buttons, tables, etc. are all specific versions of Views.
XML: Extensible Markup Language is an easy language to learn that is used for many different kinds of programs. Some people don't consider it to be a real programming language, in part because of its simplicity. It does, however, make writing Android apps very smooth for beginners.