Chapter 2: User Interface - Part 3
At this point, you've probably noticed something disconcerting. Your preview window should look something like this:
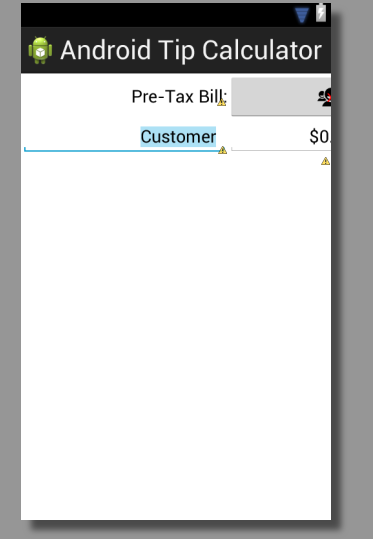
The addDinerButton is all stretched out, and the table doesn't even fit on the screen. This is because of the way Android automatically resizes views. To fix this, we need to align the addDinerButton and addButton1. See below.
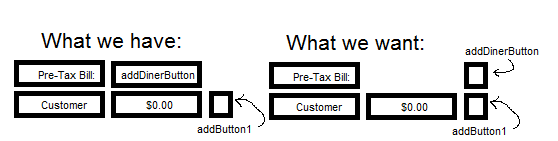
So we need to move the addDinerButton over one column. However, you'll notice that there is no column property. That's because the Graphical Layout tab does not show every property a View can possibly have. To set the column, we have to switch to the XML tab. Let's do that, and find the code for addDinerButton. Add this line to the end of the addDinerButton block of code:
android:layout_column = "2"
This moves addDinerButton to the third column of the table. Note that the layout_column property assumes the first column is column 0. The addDinerButton should no longer be stretched out, and it should be aligned with addButton1.
We still have to make our app fit on the screen horizontally. For now, let's stick with the XML tab, to practice more with the code side of things. Find the code block that refers to mainTable. Set the layout_width to fill_parent, and the layout_height to wrap_content. Fill_parent means the View will try to expand to fill the View it's residing in (the parent View). Wrap_content means the View will attempt to just big enough to enclose its content. While we're here, add the following four lines to the mainTable code block:
android:paddingTop = "5dp"
android:paddingLeft = "5dp"
android:paddingRight = "5dp"
android:paddingBottom = "5dp"
This will give us a border of space around mainTable, which makes it look nicer. Here, dp stands for density-independent pixel. Using dp instead of pixels (px) to measure distances is great because it will look more or less the same across devices of different resolutions. Next, add the following lines to mainTable:
android:shrinkColumns = "0, 1"
android:stretchColumns = "0, 1"
The 0s in these lines of code refers to column 0 (i.e. the first column). The 1s mean true. So what the whole thing means is, set the first column to be shrinkable and stretchable. Now, the first column will shrink if it makes the table go off-screen, and stretch to fill the screen if the table doesn't take up the whole screen. If you go back to the Graphical Layout tab and look at the preview, we see that our app fits completely within the screen again. What's cool is Android seems to have adjusted the size of both the first and second columns, to make them equal in size, which results in an aesthetically pleasing effect.
Glossary
Column: Normally, Views in a row of a TableLayout have consecutive columns. That is, if you put two Views in a row, the first View will go in the first column, and the second View will be in the second. However, you can change this by setting the column property. The first column corresponds with a value of 0 (second is 1, third is 2, etc.).
DP: A density-independent pixel is a unit of measurement that tries to compensate for the different resolutions of Android devices. A pixel will have a different physical, real-world size depending on the resolution of a device. However, a density-independent pixel should look very similar across most Android devices. Using dps over regular pixels is strongly suggested in almost all cases, as it provides for a uniform user experience across different devices.
Parent View: When we are talking about View hierarchy, the parent View of a View is the View that the View is inside. Okay, let me reword that. If we have a TextView inside of a TableLayout, then the TableLayout is the parent View of the TextView, and the TextView is the child View of the TableLayout. Further, if the TableLayout is itself inside a ScrollView (making it a child of the ScrollView), then the ScrollView is also a parent of the TextView. That is, parent-child relationships are inherited. As you can see, such a setup would make the TableLayout both a parent and a child View, which is very common.