Chapter 4: Extra Features - Part 2
Alright then, the first thing I think I want to implement is a random name generator. Right now, every time we create a new Diner, his default name is Customer. That's a little boring. Let's make it so the default name is a randomly selected name.
The basic idea is to have a list of names, and then, when a new Diner is created, set the appropriate EditText and TextView to a randomly chosen name from that list. That means we need to learn how to implement randomization in Java. But first, let's get a list of names going.
You may think you already know how to do this. And you do: with an ArrayList. However, there's actually something simpler than that we can use here. We can use an Array. An Array is like an ArrayList, but much lower tech. It doesn't have all the cool methods ArrayLists have. As such, an Array is very much fixed. You can't add or take out elements from it. It also does not require an import, since it's part of Java's base functionality. There are plenty of other differences too, but I'll leave you to research that yourself. The main benefit of using an Array over an ArrayList is that computers deal with Arrays a lot faster than ArrayLists. In retrospect, I probably should have gone over Arrays before ArrayLists. That's what most intelligent tutorial writers do. Oh well. In the Diner class, declare this Array:
public String[] randomNames = {"Alexander", "Gandhi", "Bonaparte", "Lincoln", "Caesar", "Washington", "Picasso", "Michelangelo", "Aristotle", "Illidan"};
The syntax is pretty intuitive. First, we have the type of element the Array will hold, in this case strings. Then we have []. Then we have the name we want to give this new Array, which is randomNames in this case. I decided to pick some famous historical figures. If you're going to have lunch with people, you could do a lot worse than these guys. Finally, we have a list of strings separated by commas inside of these {}.
Okay, now that we have this Array, let's put it into action. At the end of the Diner constructor, put this:
Random rand = new Random();
etName.setText(randomNames[rand.nextInt(randomNames.length)]);
setName();
You're also going to need to import this:
import java.util.Random;
Let's dissect this. We created a Random object called rand. A Random is an object we can use to generate random numbers. In order to use it, we need to instantiate it first, which we do with new Random();. We then set etName's Text to randomNames[rand.nextInt(randomNames.length)].. Okay, so that's a little complicated. First, you need to know that to access an element in an Array, you use this notation: ArrayName[Element Position]. So if you wanted to the third name in randomNames, you'd use: randomNames[2]. Like most things in Java, the first element in an Array is at position 0.
Of course, we don't have a simple integer here. We have rand.nextInt(randomNames.length). This is calling the nextInt method of rand, the Random object. The nextInt method will generate a random integer between 0 and the integer before the argument it is passed. So rand.nextInt(5) will give a random integer between 0 and 4. Instead of passing an integer to nextInt, we're passing randomNames.length. Like ArrayLists, Arrays also have a length attribute that tells us how many elements are in it. However, unlike ArrayLists, we don't use a method to access it. Instead, we access the attribute directly, which is why there are no () after the word length. This is similar to how we can access the total attribute of a Diner using Diner.total.
So since we're passing the length of randomNames, it will evaluate to the integer 10 in this case. 10 is then passed to nextInt, which will generate an integer between 0 and 9. That number will then be the position in randomNames that we want to use to get an random name. Once we have that, we set the Text of etName to that name. Once finished, we use the setName method to get the name to change in the split bill portion of the app as well.
Also, since we're setting the name like this, we don't need to keep putting the word Customer into EditTexts when we create new Diners. So quickly go back to MainActivity, and remove this line from addDinerButton's part of the onClick method:
et1.setText("Customer");
That line is no longer necessary. Ok, let's test. At first, all seems well.
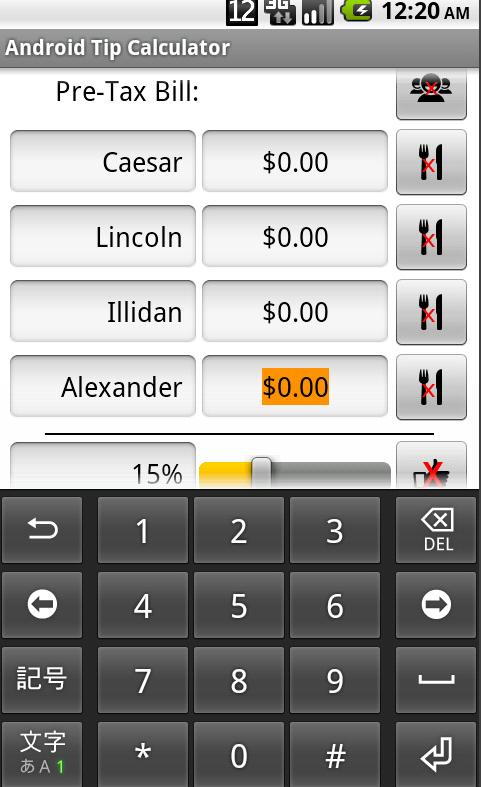
The names are indeed showing up properly. But as programmers, we must be ever vigilant for bugs. As we scroll down to check the split bill portion of the app, we run into a problem. While the names are working as intended, the totals are a little messed up.
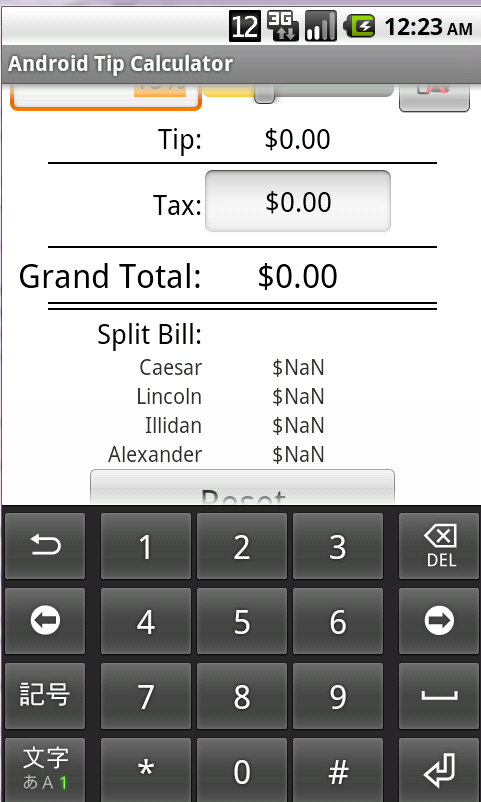
Great, a new bug. Let's take a look at this in the next part of the tutorial.
Glossary
Array: An Array is a way to group a set of similar elements together. It is a simpler version of an ArrayList.
nextInt(int n): The nextInt method randomly generates an integer between 0 and the argument it gets passed.
Random: The Random object is a random number generator.