Chapter 3: Java - Part 22
At this point, we're done with the tip calculation and grand total calculation. We just need to incorporate tax, and we'll be done with all the major parts of our app. Stay strong, we're getting there. Declare these variables:
private double tax;
private EditText taxDollar;
We'll use a double called tax to keep track of the tax. We also need access to the EditText for tax, which we'll call taxDollar. Assign as usual in onCreate.
tax = 0.0;
taxDollar = (EditText) findViewById(R.id.taxDollar);
Still in onCreate, set a focus listener to taxDollar.
taxDollar.setOnFocusChangeListener(this);
Now go to the onFocusChange stuff. Below the code for tipPercent, but above the code for the order EditTexts, insert a new conditional block code, such that onFocusChange looks like this:
if (v == tipPercent && hasFocus == false) {
...
} else if (v == taxDollar && hasFocus == false) {
tax = Double.parseDouble(((EditText) v).getText().toString().replace("$", "").replace(",",""));
((EditText) v).setText("$" + String.format("%,.2f", tax));
calcGrandTotal();
} else {
...
}
The first ... represents the code that runs when tipPercent loses focus. The second ... represents the code that runs for the order EditTexts. In between, we have an else if block. This isn't really a new concept. Basically, a simple if-else system has an if block and an else block. However, you can stretch out the system using else if. The system has to start with an if block, and can end with one and only one else block. However, you can have as many else if blocks in between those as you want. So here, whenever something gains or loses focus, onFocusChange checks if it's tipPercent. If not (else), it checks if it's taxDollar. If not, it goes on to the else block. Here's a complimentary infographic.
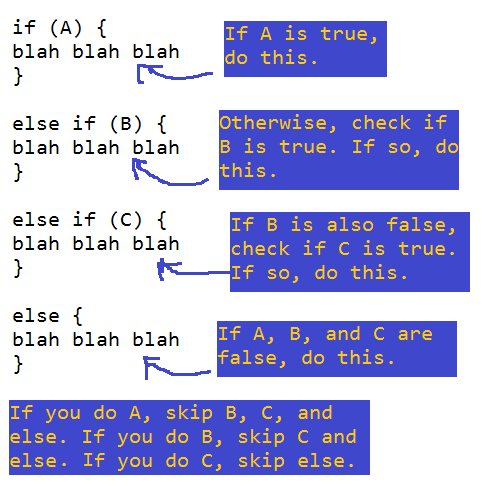
If the thing that triggered onFocusChange is taxDollar, and if it lost focus rather than gained it, we set the tax variable equal to whatever was inside v (which would be taxDollar). As always, we need to cast v as an EditText to get its Text. Once we've set the tax variable, we go back and set the Text for v to tax, but with proper formatting. Yes, we're basically setting tax to taxDollar, then taxDollar back to tax, in order to format it. Finally, we call calcGrandTotal. Of course, calcGrandTotal doesn't currently include tax in its calculations, so lets fix that. Go to the definition for calcGrandTotal, and change the last line that sets the Text of textGTotal to this:
textGTotal.setText("$" + String.format("%,.2f", grandTotal + totalTip + tax));
Now the grand total TextView will take tax into account. Great, go ahead and test now.
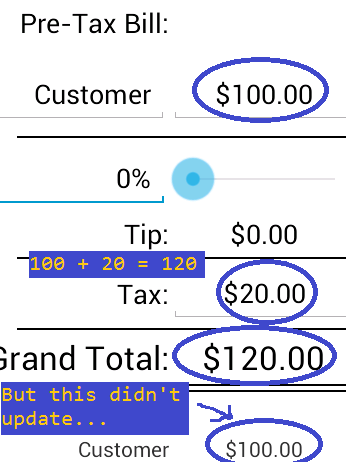
Well, that's pretty good. Not perfect though. While textGTotal does show the correct grand total, including tax, the split bill doesn't include a Diner's portion of the tax. Let's work on that next.