Chapter 3: Java - Part 5
One useful way to fix this is to keep track of how many diners there are in our app. But how do we do that? We could simply keep track of a number that increases each time the user creates a new diner. That would work. But this is an excellent opportunity to give our app some organization. Let me explain. What is the core thing that our app keeps track of? It's the diners. But what is a diner, as far as our app is concerned? It is, collectively, a thing that includes the EditTexts for his name and the things he ordered, his ImageButton that adds more orders for him, and the two TextViews that have his name and total portion of the bill, down in the bill splitting part of our app. Those are all the objects in our app associated with any one particular diner. Now, if we kept track of each diner in the form of this collection of objects, we would have a pretty good way of organizing our app's information, internally. And then, we could just count how many diner objects we have, and use that number to find out which row to add row2 to.
But of course, a diner object, as we have just described it, does not exist anywhere else in the world. We can't just import it from somewhere. That would be too easy. Instead, we're going to have to make this object ourselves. And how do we make an object in programming? As you know, we start with the blueprints: a class. Let's make a class.
Right-click the app's package in the src folder in the Package Explorer window, and under New, find and click Class. You can leave everything as is in the new window that pops up, just make the Name field say Diner. Hit the Finish button, and look back in the Package Explorer. We now have a Diner class. Open it up so we can edit it.
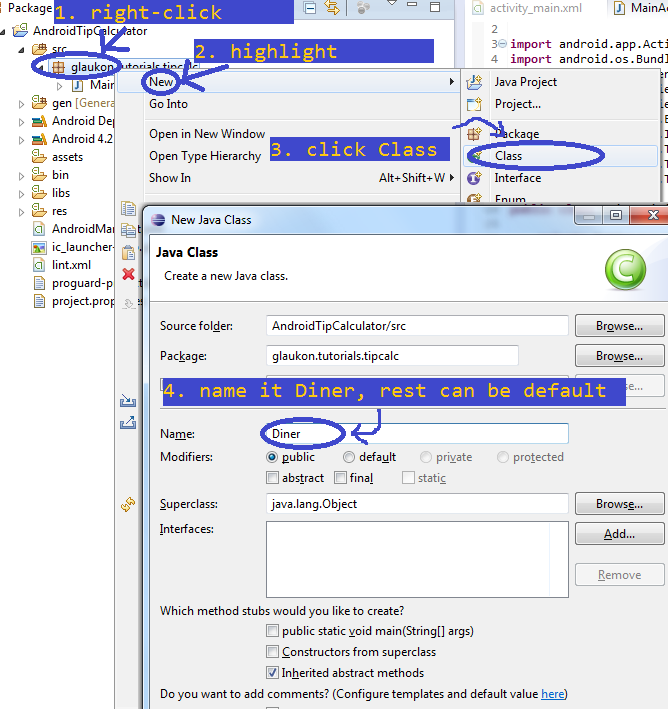
Our new class doesn't have a lot to it right now, just some package info, and an empty declaration. Let's spruce it up a bit. To that end, let's make a constructor. In programming, a constructor is a method that runs when an object is created. The code inside the constructor 'constructs', or builds, the object. It's a little like the onCreate method for Activities (but not quite, for reasons that aren't too important here). To create a constructor, simply make a method in the Diner class called Diner. A constructor always has the same name as the class it is constructing. Make your Diner class look like this:
public class Diner {
public Diner() {
}
}
The method called Diner, inside our Diner class, is what gets called when we instantiate a Diner object from this class. Let's fill that method with code that will create a Diner object, as we defined it earlier. A Diner object has the EditTexts with a name and order amounts, an ImageButton for more orders, and two TextViews with a name and the portion of the bill the corresponding diner is responsible for. So let's add these things as arguments to our Diner method, and make use of them. Change the diner class to look like this:
public class Diner {
public EditText etName;
public EditText etFirstOrder;
public ImageButton ibAddOrder;
public TextView tvName;
public TextView tvSplitBill;
public Diner(EditText et1, EditText et2, ImageButton ib, TextView tv1, TextView tv2) {
etName = et1;
etFirstOrder = et2;
ibAddOrder = ib;
tvName = tv1;
tvSplitBill = tv2;
}
Of course, make sure you import whatever you need to. Note that since this is a new class, we need to re-import everything we want to use.
import android.widget.EditText;
import android.widget.ImageButton;
import android.widget.TextView;
So, here we declared 5 variables. We want them to hold the 5 elements that make up a brand new Diner: 2 EditTexts, an ImageButton, and 2 TextViews. These are the things that get created automatically when our user hits addDinerButton. Then, we change the Diner constructor to accept these objects as arguments. Inside the method, we assign the variables to the objects that were passed in. So, whenever we want to create a new Diner object to organize all the elements related to one particular diner, we simply pass the 5 objects that are associated with a new Diner, and our constructor will set those 5 things to the etName, etFirstOrder, ibAddOrder, tvName, and tvSplitBill of a new Diner object. Perhaps it would be best to show you how this works, rather than just telling.
Before going on, some of you with previous programming experience might take issue with making all the variables here public. I agree. But I don't want to get into encapsulation in this tutorial. It's not vital for a beginner to understand, and it isn't necessary for a project of this simplicity. Anyway, go back to MainActivity. At the end of addDinerButton's onClick code block, add this line:
Diner diner = new Diner(et1, et2, ib, tv1, tv2);
This line declares a variable called diner. It is meant to hold an object of the type Diner. In the same line, we assign it to a new Diner object we instantiate. To instantiate it, we pass 5 things to the constructor of Diner. The 5 things are et1, et2, ib, tv1, and tv2. These are the 5 objects our code creates when addDinerButton is clicked. Here, we create a Diner object to tie all 5 objects together into one organizational unit that represents a diner. Called Diner. Ya.
Glossary
Constructor: A constructor is a special kind of method within a class that is used to create objects from that class. When you instantiate an object, the constructor method is called.