Chapter 3: Java - Part 17
First, let's edit the Diner class's updateTotal method a little, so that it accounts for the tip. In the Diner class, change the updateTotal method declaration to this:
public void updateTotal(EditText toBeUpdated, double tip) {
We gave updateTotal another parameter, which is the tip. Now we'll make it use the tip in its calculation. Find the line in updateTotal that sets the Text for tvSplitBill and replace it with this:
tvSplitBill.setText("$" + String.format("%,.2f", total * (1+tip)));
Now, instead of just setting the tvSplitBill of a Diner to its total, we set it to the total multiplied by 1 plus the tip. Of course, having done this, we need to go and change MainActivity again. So find the line in MainActivity that has the updateTotal method, and change it so that it passes 2 arguments instead of just the one.
dinerList.get(i).updateTotal((EditText) v, tipPercentValue);
Let's test again.
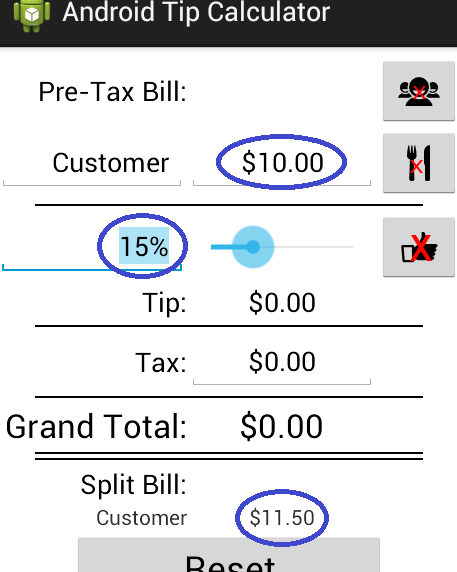
As you can see, after we edit any order EditText, our app now calculates a Diner's split bill including the tax. Still, if you try and move tipSlider around at this point, the amounts in the split bill portion of the app won't update in real-time. That's because we only calculate when the user finishes editing an order EditText.