Chapter 3: Java - Part 18
Let's now make it so the amounts will update as the user is manipulating tipSlider. Go to the onProgressChanged block, and append this for loop to the end of it.
for (int i = 0; i < dinerList.size(); i++) {
dinerList.get(i).updateTotal(tipPercentValue);
}
This for loop, which we are very familiar with, runs through all the Diners in dinerList. For each Diner, it calls its updateTotal method. However, you'll notice we only pass 1 argument here, tipPercentValue. We just finished editing updateTotal to take 2 arguments, so Eclipse is upset at us. Let's go back to the Diner class and fix things up a little. Underneath the updateTotal method, add this method:
public void updateTotal(double tip) {
updateTotal(etFirstOrder, tip);
}
We now have two methods called updateTotal in our Diner class called updateTotal. You may have assumed that this is not possible. While that would be a very reasonable assumption, in fact, it is very possible to have multiple methods with the same name. Moreover, you've already seen examples of this in action. For instance, I said the addView method can have 2 arguments, but the second one is optional. If you pass a second argument, the View is added at that position. However, if you leave the second argument out, the View is added as the first View. What this means is that there are actually 2 versions of addView. One version only has one parameter, and one has 2 parameters. The computer automatically picks the correct version based on what and how many argument(s) you pass. This process of creating multiple methods with the same name but different parameters is called overloading a method.
Here, we're overloading updateTotal. We want to have 2 versions. The first version is when an order EditText just got edited. That version takes both the EditText, as well as the tip as arguments. This is so we can format the EditText that was just updated. The second version only has one parameter, the tip. This is the one we use when the user only changed the tip, and not any particular order EditText. Inside this version, we simply call the first version of updateTotal, and pass it etFirstOrder as the first of the 2 arguments. We know that every Diner has an etFirstOrder, so basically, whenever we use updateTotal with just the tip as an argument, this version of the method will transfer that method over to the first version of updateTotal, with etFirstOrder as a default argument. This way, our code we wrote earlier in MainActivity with just one argument for updateTotal will work. There are other ways of getting this to work, but I felt like it was a good time to introduce method overloading. Let's test the app.
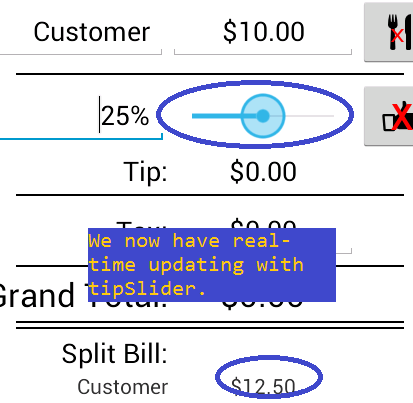
Put some orders in, and then slide tipSlider around. The bill splitting part of the app should update with tip being calculated properly in real-time. Congrats! I'd say we're probably more than half done with this tutorial now! (Yay?) I think I mentioned this earlier, but if not, remember, the AVD is supposed to lag. It's slow. Real Android devices will run your apps much faster.
Glossary
Overloading: Overloading refers to creating multiple methods with the same name, but different parameters.