<TableRow
android:id="@+id/tableRow14"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<Button
android:id="@+id/resetButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="50dp"
android:layout_marginRight="50dp"
android:layout_span="3"
android:text="Reset"
android:textSize="24dp" />
</TableRow>
<TableRow
android:id="@+id/tableRow15"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/fortuneTextBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_span="2"
android:gravity="center"
android:text="[Insert Fortune Here]" />
<ImageButton
android:id="@+id/fortuneButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/fortune" />
</TableRow>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/bg"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableLayout
android:id="@+id/mainTable"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:paddingBottom="5dp"
android:paddingLeft="5dp"
android:paddingRight="5dp"
android:paddingTop="5dp"
android:shrinkColumns="0, 1"
android:stretchColumns="0, 1" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right|center_vertical"
android:text="Pre-Tax Bill:"
android:textAppearance="?android:attr/textAppearanceMedium" />
<ImageButton
android:id="@+id/addDinerButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_column="2"
android:src="@drawable/adddiner" />
</TableRow>
<TableRow
android:id="@+id/tableRow2"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<EditText
android:id="@+id/firstCustomer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:ems="10"
android:gravity="right|center_vertical"
android:inputType="textPersonName"
android:selectAllOnFocus="true"
android:text="Customer" />
<EditText
android:id="@+id/amount1of1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:ems="10"
android:gravity="center"
android:inputType="numberDecimal"
android:selectAllOnFocus="true"
android:text="$0.00" >
<requestFocus />
</EditText>
<ImageButton
android:id="@+id/addButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/additem" />
</TableRow>
<TableRow
android:id="@+id/tableRow3"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="1dp"
android:layout_marginBottom="3dp"
android:layout_marginLeft="25dp"
android:layout_marginRight="25dp"
android:layout_marginTop="3dp"
android:layout_span="3"
android:background="#000"
android:text="" />
</TableRow>
<TableRow
android:id="@+id/tableRow4"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<EditText
android:id="@+id/tipPercent"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:ems="10"
android:gravity="right|center_vertical"
android:inputType="number"
android:selectAllOnFocus="true"
android:text="15%" />
<SeekBar
android:id="@+id/tipSlider"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:max="50"
android:progress="15" />
<ImageButton
android:id="@+id/roundButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/round" />
</TableRow>
<TableRow
android:id="@+id/tableRow5"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right|center_vertical"
android:text="Tip:"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/tipDollar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="$0.00"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<TableRow
android:id="@+id/tableRow6"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="1dp"
android:layout_marginBottom="3dp"
android:layout_marginLeft="25dp"
android:layout_marginRight="25dp"
android:layout_marginTop="3dp"
android:layout_span="3"
android:background="#000"
android:text="" />
</TableRow>
<TableRow
android:id="@+id/tableRow7"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right|center_vertical"
android:text="Tax:"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/taxDollar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:ems="10"
android:gravity="center"
android:inputType="numberDecimal"
android:nextFocusDown="@+id/amount1of1"
android:nextFocusRight="@+id/amount1of1"
android:selectAllOnFocus="true"
android:text="$0.00" />
</TableRow>
<TableRow
android:id="@+id/tableRow8"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView6"
android:layout_width="wrap_content"
android:layout_height="1dp"
android:layout_marginBottom="3dp"
android:layout_marginLeft="25dp"
android:layout_marginRight="25dp"
android:layout_marginTop="3dp"
android:layout_span="3"
android:background="#000"
android:text="" />
</TableRow>
<TableRow
android:id="@+id/tableRow9"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right|center_vertical"
android:text="Grand Total:"
android:textAppearance="?android:attr/textAppearanceLarge" />
<TextView
android:id="@+id/textGTotal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:gravity="center"
android:text="$0.00"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<TableRow
android:id="@+id/tableRow10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView8"
android:layout_width="wrap_content"
android:layout_height="1dp"
android:layout_marginBottom="1dp"
android:layout_marginLeft="25dp"
android:layout_marginRight="25dp"
android:layout_marginTop="3dp"
android:layout_span="3"
android:background="#000"
android:text="" />
</TableRow>
<TableRow
android:id="@+id/tableRow11"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView9"
android:layout_width="wrap_content"
android:layout_height="1dp"
android:layout_marginBottom="3dp"
android:layout_marginLeft="25dp"
android:layout_marginRight="25dp"
android:layout_marginTop="1dp"
android:layout_span="3"
android:background="#000"
android:text="" />
</TableRow>
<TableRow
android:id="@+id/tableRow12"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView10"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:text="Split Bill:"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<TableRow
android:id="@+id/tableRow13"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textSplit1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="right"
android:text="Customer" />
<TextView
android:id="@+id/textSplit1Dollar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="$0.00" />
</TableRow>
<TableRow
android:id="@+id/tableRow14"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<Button
android:id="@+id/resetButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="50dp"
android:layout_marginRight="50dp"
android:layout_span="3"
android:text="Reset"
android:textSize="24dp" />
</TableRow>
<TableRow
android:id="@+id/tableRow15"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/fortuneTextBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_span="2"
android:gravity="center"
android:text="[Insert Fortune Here]" />
<ImageButton
android:id="@+id/fortuneButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/fortune" />
</TableRow>
</TableLayout>
</ScrollView>
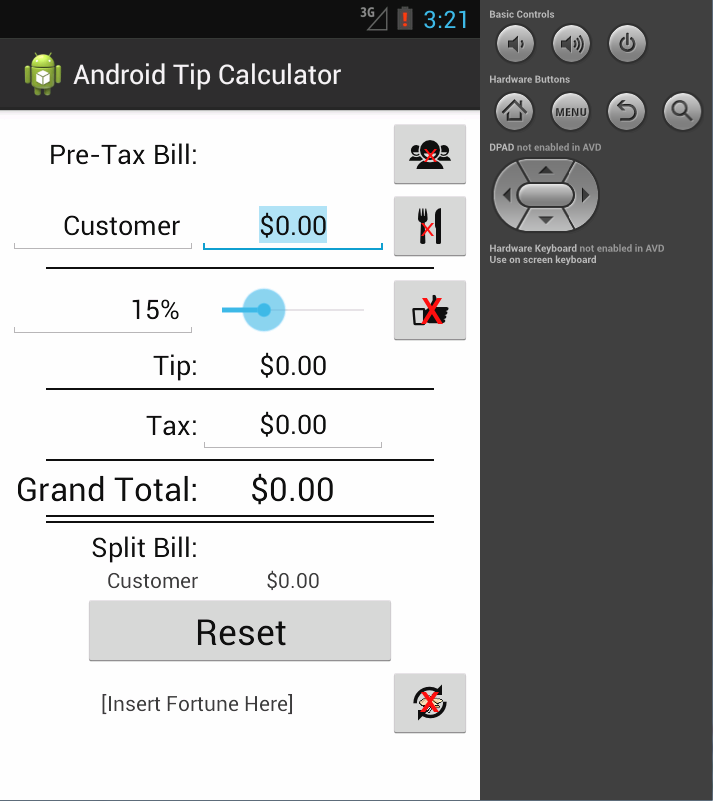