Chapter 3: Java - Part 1
Are you back? Did you take a good, long break? Good, because it gets harder from here. Before going on, you may want to practice a little more with XML. One exercise you can try is to delete everything we created, and try to create the UI from scratch again with straight XML. It's great for familiarizing yourself with the syntax. Anyway, let's get to the Java. Open up your Java file, which we called MainActivity.java. It's in the src folder, in the glaukon.tutorials.tipcalc package, if you followed me exactly.
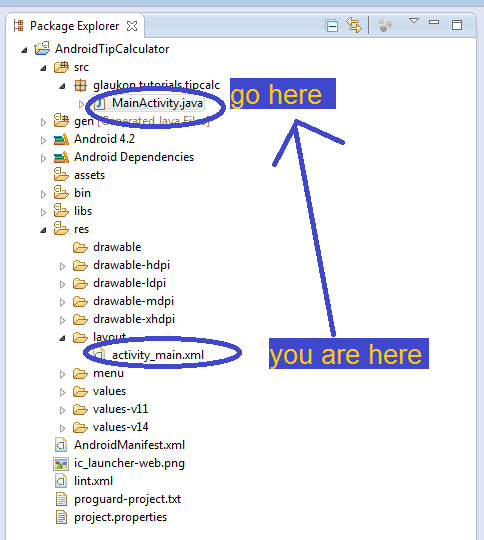
Right then, as you can see, Eclipse created some code for us. Let's go over it. Computers generally read code from the top, and then go down, starting with the first line. The first line tells us (and others) where our project is:
package glaukon.tutorials.tipcalc;
There's a new keyword here: package. Packages are how Java organizes resources. They're kind of like directories on your computer. Knowing the package of a project is important if you want to use the code from that package. If another coder wanted to use the code you make, he would need to know the package info. Also, note that the line ends in a semicolon. Most lines in Java end in a semicolon (it tells the computer the line has ended). I will point out the ones that don't. After that line, we have the import statements:
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
If you only see one import statement, click the + icon next to it to expand the import code block. A statement in programming is a line that tells the computer something. Import statements tells the computer where to find some code. Programmers often use code that other programmers wrote. To do this, they import code from other projects. Some projects are designed specifcally for others to import. These are called libraries. These 3 lines tell the computer to import 3 parts of the Android library. We use these parts in the lines ahead. By importing them, the computer now knows where to look for code about Bundles, Activities, and Menus. Note the use of packages in the import statements. The computer needs to know where to look. After that, we have this:
public class MainActivity extends Activity {
Oh boy. Classes. The big one. The head honcho of objected-oriented programming (OOP). I've been delaying explaining this part for as long as possible. But there's no avoiding it now. What is a class? You can think of it as the blueprints for an object. A class defines a hypothetical thing that has certain attributes and methods. That thing doesn't actually exist yet. But we can use the blueprints, the class, to create an object. This is called instantiating an object from a class. You can create/instantiate many objects from one set of blueprints.
For instance, say we have blueprints for a cat object. These blueprints say a cat is something with a height, a weight, an age, and is something that can walk, and meow, and eat. These blueprints may have default values for the attributes, and have default definitions for the methods, although it isn't necessary. Using these blueprints, we can create many cats. But not all cats are instantiated equal. Some cats will be shorter/taller, some will be skinnier/fatter, and some will be younger/older. They may also have different ways of walking, meowing, and running. These cats are all objects created from the cat class. They have the same attributes and methods. But the values and definitions of these attributes and methods don't have to be the same. So ya, class = blueprints, any questions?
Well, I'm sure you have many. These aren't concepts you learn overnight after reading a novice level programming tutorial written by a bad programmer. But sometimes it helps to keep pushing forward and get more information that will help you process the current information. So let's push forward. This line is a line that declares something. We know this because of the keywords and punctuation being used. The keyword public refers to whether or not other objects can easily and directly interact with and manipulate objects created from this class. If it's public, then the answer is yes. Generally, having too many things that are public in your program is bad. It's less secure, and harder to debug. However, since our program is so small, we won't worry about that. We'll talk more about the public keyword in another tutorial.
The keyword class says we are declaring a class. MainActivity is the name of the thing we're declaring. The keyword extends basically means 'inherits from'. Next, Activity is the class we're extending, or inheriting from. Note that we're only able to use the Activity class in the code because we imported the Activity code from the Android library earlier.
You remember what inheriting means, right? It means we're getting all the attributes and methods from the Activity class. And finally, that fancy bracket at the end of the line (instead of a semicolon) means we're declaring something. Everything between the fancy brackets are part of the declaration. When we're talking about classes, declaring refers to the process of setting the attributes and methods of that class. So what does this whole line mean? We're declaring a class called MainActivity. It is public, so other objects can communicate with the objects created from this class. It extends the Activity class, and so has all the attributes and methods of Activities. Now, what is an activity?
I like to think of Activities as windows, like on your computer. These windows will have things inside of them that the user can usually do stuff to. You know, text, images, buttons, and such. So, how are we declaring MainActivity? That is, what are we saying about it?
@Override
protected void onCreate(Bundle savedInstanceState) {
One thing we're going to say is that it overrides the onCreate method. The onCreate method is something we inherited from the Activity class. It's a method that automatically runs when an Activity is started, and sort of sets up an Activity for use. Overriding refers to the act of replacing a method we inherited with our own code. So basically, our MainActivity class inherited a method from Activity, but we want to make it more customized, so we're going to override what onCreate normally does in Activities, and tell it to do something more niche. That's right, we can replace methods we inherit. Again, not all cats are the same. They don't all walk the same, so if we have a cat that doesn't walk like all the other cats, we can create a new class that inherits from the base cat class, but overrides its default walk method and gives it something more specific. We're going to ignore the protected keyword and (Bundle savedInstanceState) for now since they're not too important. The void keyword refers to onCreate's return type. Really quickly, methods can do stuff and tell you stuff. Returning has to do with telling you stuff. The onCreate method usually does stuff, and doesn't tell us anything, so it returns void, which kind of means 'nothing'. Again, not too important, so let's move on.
Anyway, here we are overriding onCreate with the stuff that's inside the next set of fancy brackets. What do we replace it with?
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
We have another new keyword: super. The super keyword means run the parent class's version of the following code. So super.onCreate means run the parent class's version of onCreate. Let's ignore the part about savedInstanceState for now, we won't need to know about it for this app. The parent class refers to the class we inherited from, or extended, which is Activity in this case. After we run the Activity class's onCreate method, we use the method setContentView. The setContentView method is another method we inherited from Activity. It is a method that takes an argument. Sometimes a method needs information in order to do what it needs to do. We can give a method information by giving, or passing, it an argument (or multiple arguments). In this case, we pass the setContentView method an argument of R.layout.activity_main. This tells setContentView where to find our XML document we made that has all our UI information. The setContentView method then finds that XML document, and uses it to create our UI. This process of using an XML document to create the UI is called 'inflating' the UI using XML. So, to re-cap, we're replacing the default Activity version of onCreate (which is a method that is run when an Activity is created) with our own. Our own version first runs the default version, but then also inflates our UI.
Wow, we're barely getting started and I already feel exhausted. That's programming for you. After this override block (blocks of code are marked by empty lines and help make code more readable), we have this:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
This is very similar to the last override block (note that these are also method declarations). Here, we override onCreateOptionsMenu. The onCreateOptionsMenu method is code that runs when you hit the options button. Every Android phone has an options button. It's public and returns a Boolean. We don't care about that. The next line starts with two foward slashes. Any time you have two forward slashes in Java, the computer will ignore the rest of the line after those slashes. This allows you to put comments in your code without confusing the computer. It's good to get into the practice of putting lots of comments in your code. It keeps you from being as confused when you're reading it over. It's also useful if you plan to share your code. After the comment, we have the declaration for onCreateOptionsMenu we want to use instead of the default one. The declaration says to inflate the options menu with the XML document in the menu folder called activity_main. By the way, the R.menu here doesn't actually refer to the menu folder directly, and has to do with the automagically generated R.java file in the gen folder. Don't worry about it, it's something Eclipse does in the background to make our lives easier, and not too important to understand right now. Anyway, since we never edited the menu XML, our app's menu is the default menu Eclipse made for us. Basically, it's just one item that says settings, and when you click it, nothing happens. You can run the app in an AVD to see for yourself. After that, the method returns true. You can ignore that part. And again, the last fancy bracket marks where the declaration ends.
Right then, so that's it. That explains all the code that Eclispe gave us for free. We defined our package, imported some stuff we needed, defined something we called MainActivity, which inherits from Activity, and overrode two of the methods it inherited. In doing so, we inflated our UI and options menu from XML documents in our package. Awesome. This is the same basic code that Eclipse always generates for us, so now that we understand it (right?), we don't have to waste time on it in the next tutorial.
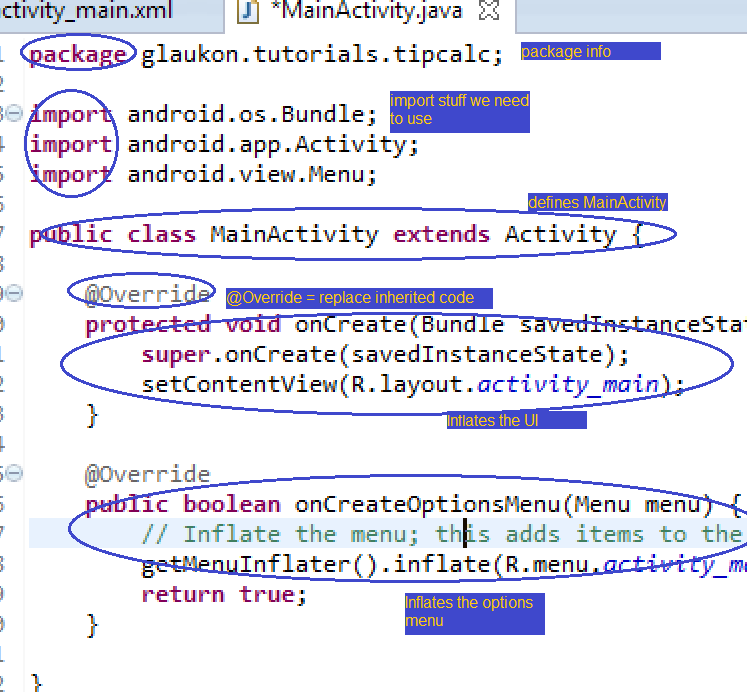
Before moving on, you may be wondering what part of the code here actually tells MainActivity to run. I mean, we told it to do stuff when it's created, but what creates it? Basically, Eclipse automatically set this Activity as the special Activity that Android instantiates automagically when we launch the app. We can change this if we want, but we won't need to here. So what happens is, the user launches our app, Android sees MainActivity is the special, default class, so it creates an object using the MainActivity class as the blueprints. This MainActivity object then has the onCreate method, which tells it to inflate the UI when Android instantiates it. And thus, our app has a UI when we run it in the AVD. That's how it all works together.
Glossary
Activity: An Activity object is kind of like a window. You can do stuff in Activities, like click on buttons or look at text.
Argument: An argument is something you pass to a method to give it more information in order to complete the task it is designed to complete.
Class: A class is like a set of blueprints for an object. You instantiate objects from a class. Many objects can be made from the same class.
Comments: Comments are things that the computer ignores in your code, but that you can read. They're helpful in that they remind you what certain code blocks do, and make your code more accessible to others.
Declare: Declaring essentially refers to the act of telling the computer something exists, as well as a few facts about the thing. You can declare classes, methods, and variables. To declare a class means to set up all the methods and variables for a class. To declare a method means to define what a method should do.
Import: The import statement allows you to use code from outside your project's package.
onCreate(Bundle savedInstanceState): The onCreate method is called as soon as an Activity object is created.
onCreateOptionsMenu(Menu menu): The onCreateOptionsMenu method is called when the user accesses the options menu.
OOP: Object-oriented programming is a programming paradigm that uses objects as integral building blocks of programs.
Override: Overriding a method refers to replacing a method definition that was inherited with custom code.
Packages: Packages are how Java organizes your project. From a simplistic perspective, a package is kind of like a directory on your computer, in the sense that it describes the location of your project. Sort of. You may want to check out the Wikipedia page for namespaces too.
Parent Class: If class A inherits from class B, then class B is the parent of class A and class A is the child of class B. Class B is also the parent of whatever classes inherit from class A, and class A is also the child of whatever classes class B inherits from.
Pass: Passing refers to the act of giving arguments to a method.
return: The return keyword says to tell the computer something. Some methods will return a value after it is finished running, and that lets you use the value that is returned to do other stuff.
setContentView(View view): The setContentView method sets the UI of an Activity object based on the XML file it's passed as an argument.
Statement: A statement is just a line in programming that tells the computer something.
super: The super keyword is used when you want to access the inherited version of a method.
void: The void keyword is used when you want to define a method that does not return anything.